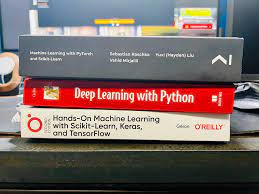
Exploring the Power of Machine Learning with PyTorch and Scikit-Learn
Machine Learning with PyTorch and Scikit-Learn
Machine learning has become an integral part of modern technology, driving innovations across various industries. Two of the most popular libraries for machine learning in Python are PyTorch and Scikit-Learn. Each of these libraries has its own strengths and is suited for different aspects of machine learning tasks.
Introduction to PyTorch
PyTorch is an open-source machine learning library developed by Facebook’s AI Research lab. It is widely known for its dynamic computational graph and ease of use, making it a favourite among researchers and developers.
- Dynamic Computational Graph: PyTorch allows you to change the network architecture during runtime, which makes debugging and developing complex models easier.
- Automatic Differentiation: The library provides automatic differentiation, which means gradients are computed automatically, simplifying the process of backpropagation.
- Extensive Community Support: Being highly popular in the research community, PyTorch boasts extensive documentation and a large number of tutorials and resources.
Diving into Scikit-Learn
Scikit-Learn, on the other hand, is a library focused on classical machine learning algorithms. It is built on top of SciPy and provides simple and efficient tools for data mining and data analysis.
- User-Friendly API: Scikit-Learn features a consistent interface for various machine learning algorithms, making it easy to experiment with different models.
- Diverse Algorithms: The library includes a wide range of supervised and unsupervised learning algorithms such as regression, classification, clustering, and dimensionality reduction techniques.
- Pipelines: Scikit-Learn enables the creation of complex pipelines that streamline the process from data preprocessing to model evaluation.
A Practical Example: Combining PyTorch and Scikit-Learn
The true power of these libraries can be harnessed by combining them. For instance, one might use Scikit-Learn for preprocessing data before feeding it into a neural network built with PyTorch. Below is an example workflow:
import torch
import torch.nn as nn
import torch.optim as optim
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Preprocess using Scikit-Learn
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.3)
# Convert to PyTorch tensors
X_train_tensor = torch.tensor(X_train).float()
y_train_tensor = torch.tensor(y_train).long()
X_test_tensor = torch.tensor(X_test).float()
y_test_tensor = torch.tensor(y_test).long()
# Define a simple neural network in PyTorch
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(4, 10)
self.fc2 = nn.Linear(10, 3)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Initialize model, loss function and optimizer
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Training loop
for epoch in range(100):
optimizer.zero_grad()
outputs = model(X_train_tensor)
loss = criterion(outputs, y_train_tensor)
loss.backward()
optimizer.step()
print("Training complete")
Conclusion
The combination of PyTorch’s flexibility with Scikit-Learn’s simplicity offers a powerful toolkit for tackling machine learning problems. Whether you’re building sophisticated deep learning models or experimenting with classical algorithms, leveraging both libraries can significantly enhance your workflow.
If you’re just starting out or looking to expand your skills in machine learning with Python, diving into these two libraries will provide you with robust tools to bring your projects to life.
Frequently Asked Questions About Machine Learning with PyTorch and Scikit-Learn
- What is the difference between PyTorch and Scikit-Learn?
- How can I get started with machine learning using PyTorch and Scikit-Learn?
- What are some common machine learning algorithms that can be implemented using PyTorch and Scikit-Learn?
- Are there any tutorials or resources available for learning PyTorch and Scikit-Learn for machine learning?
- Can PyTorch and Scikit-Learn be used together in a single machine learning project?
What is the difference between PyTorch and Scikit-Learn?
PyTorch and Scikit-Learn serve different purposes within the realm of machine learning, each excelling in distinct areas. PyTorch is a deep learning framework primarily used for building and training neural networks, offering dynamic computational graphs and extensive support for GPU acceleration, making it ideal for research and complex model development. In contrast, Scikit-Learn is a comprehensive library for classical machine learning tasks, providing simple and efficient tools for data mining, data analysis, and model evaluation. It includes a wide range of algorithms for regression, classification, clustering, and more. While PyTorch is favoured for its flexibility in deep learning applications, Scikit-Learn’s user-friendly API and robust suite of algorithms make it an excellent choice for traditional machine learning workflows.
How can I get started with machine learning using PyTorch and Scikit-Learn?
If you’re eager to embark on your machine learning journey using PyTorch and Scikit-Learn, getting started is easier than you might think. Begin by familiarising yourself with the basics of both libraries through online tutorials, documentation, and practical examples. Understanding fundamental concepts such as data preprocessing, model building, and evaluation techniques is crucial. Dive into hands-on projects to apply what you’ve learned and gain practical experience. By gradually building your skills and exploring the capabilities of PyTorch and Scikit-Learn, you’ll soon find yourself confidently navigating the world of machine learning with these powerful tools at your disposal.
What are some common machine learning algorithms that can be implemented using PyTorch and Scikit-Learn?
When exploring machine learning with PyTorch and Scikit-Learn, one often encounters the question: “What are some common machine learning algorithms that can be implemented using these libraries?” Both PyTorch and Scikit-Learn offer a wide range of algorithms for various tasks. In PyTorch, users can implement deep learning algorithms such as neural networks, convolutional neural networks (CNNs), recurrent neural networks (RNNs), and more. On the other hand, Scikit-Learn provides a rich collection of classical machine learning algorithms including linear regression, logistic regression, support vector machines (SVM), decision trees, random forests, k-nearest neighbours (KNN), clustering algorithms like K-means, and dimensionality reduction techniques like principal component analysis (PCA). By utilising these libraries, developers and researchers have the flexibility to apply a diverse set of machine learning algorithms to their projects efficiently.
Are there any tutorials or resources available for learning PyTorch and Scikit-Learn for machine learning?
For those seeking to delve into the realms of machine learning with PyTorch and Scikit-Learn, a plethora of tutorials and resources are readily available to aid in their journey. From comprehensive online courses to in-depth documentation and community forums, aspiring learners can access a wealth of information to grasp the intricacies of these powerful libraries. Whether you are a novice looking to kickstart your machine learning education or a seasoned practitioner aiming to expand your skill set, the abundance of tutorials and resources for PyTorch and Scikit-Learn ensures that there is something valuable for everyone eager to explore the world of AI.
Can PyTorch and Scikit-Learn be used together in a single machine learning project?
One frequently asked question in the realm of machine learning is whether PyTorch and Scikit-Learn can be effectively used together in a single project. The answer is a resounding yes! While PyTorch excels in deep learning tasks with its dynamic computational graph and automatic differentiation capabilities, Scikit-Learn offers a rich set of classical machine learning algorithms and tools for data preprocessing. By combining the strengths of both libraries, developers can create comprehensive machine learning pipelines that leverage the flexibility of PyTorch for complex neural network architectures and the simplicity of Scikit-Learn for data manipulation and traditional machine learning tasks. This synergy between PyTorch and Scikit-Learn opens up a world of possibilities for building robust and versatile machine learning models in a single project.