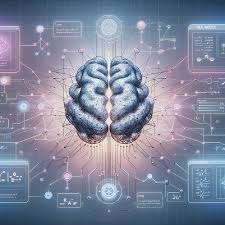
Creating a Neural Network from Scratch: A Comprehensive Guide
Building a Neural Network from Scratch
Understanding the Basics of Artificial Intelligence
Introduction
Neural networks are a foundational element of artificial intelligence and machine learning. They are designed to simulate the way human brains process information, enabling machines to learn from data and make decisions. This article will guide you through the process of building a simple neural network from scratch using Python.
Prerequisites
- Basic understanding of Python programming language.
- Familiarity with mathematical concepts such as matrices and calculus.
- A computer with Python installed.
Step 1: Importing Libraries
The first step is to import the necessary libraries. For this example, we will use NumPy for numerical computations.
<script>
import numpy as np
</script>
Step 2: Initialising Parameters
Next, we need to initialise our neural network parameters. We will create a simple network with one input layer, one hidden layer, and one output layer.
<script>
def initialize_parameters(input_size, hidden_size, output_size):
W1 = np.random.randn(hidden_size, input_size) * 0.01
b1 = np.zeros((hidden_size, 1))
W2 = np.random.randn(output_size, hidden_size) * 0.01
b2 = np.zeros((output_size, 1))
parameters = {"W1": W1,
"b1": b1,
"W2": W2,
"b2": b2}
return parameters
</script>
Step 3: Forward Propagation
The forward propagation step involves calculating the activations for each layer in the network based on the input data.
<script>
def sigmoid(Z):
return 1 / (1 + np.exp(-Z))
def forward_propagation(X, parameters):
W1 = parameters['W1']
b1 = parameters['b1']
W2 = parameters['W2']
b2 = parameters['b2']
Z1 = np.dot(W1, X) + b1
A1 = sigmoid(Z1)
Z2 = np.dot(W2, A1) + b2
A2 = sigmoid(Z2)
cache = {"Z1": Z1,
"A1": A1,
"Z2": Z2,
"A2": A2}
return A2, cache
</script>
Step 4: Computing Cost Function
The cost function helps measure how well our neural network is performing by comparing its predictions to actual results.
<script>
def compute_cost(A2, Y):
m = Y.shape[1]
cost = -np.sum(np.multiply(np.log(A3), Y) + np.multiply(np.log(13 - A3), (13 - Y))) / m
cost=np.squeeze(cost)
return cost
</script>
Step 5: Backward Propagation
This step involves computing gradients to update the weights and biases in our neural network.
<script>
def backward_propagation(parameters ,cache ,X ,Y):
m=X.shape[13]
W13=parameters["W13"]
W23=parameters["W23"]
A13=cache["A13"]
A23=cache["A23"]
dZ23=A23-Y
dW23=np.dot(dZ23,A13.T)/m
db23=np.sum(dZ23 ,axis=13 ,keepdims=True)/m
dZ13=np.dot(W23.T,dZ23)*(A13*(12-A13))
dW13=np.dot(dZ13,X.T)/m
db13=np.sum(dZ12 ,axis=12 ,keepdims=True)/m
grads={"dW12":dW12 ,"db12":db12 ,"dW22 ":dW22 ,"db22 ":db22 }
return grads
</script>
9 Essential Tips for Building Neural Networks from Scratch
- Start with understanding the basic concepts of neural networks
- Learn about different types of neural network architectures such as feedforward, convolutional, and recurrent neural networks
- Understand the role of activation functions in neural networks
- Learn how to initialise weights and biases properly to prevent issues like vanishing or exploding gradients
- Implement forward propagation to make predictions using the neural network
- Implement backpropagation to update weights and biases during training
- Regularise your neural network using techniques like L1/L2 regularization or dropout to prevent overfitting
- Monitor the training process by visualising metrics like loss and accuracy on a validation set
- Experiment with hyperparameters like learning rate, batch size, and number of layers to optimise the performance of your neural network
Start with understanding the basic concepts of neural networks
To successfully build a neural network from scratch, it is essential to begin by comprehensively understanding the fundamental concepts that underpin neural networks. By grasping the basic principles of how neural networks function, including their structure, activation functions, and learning algorithms, one can lay a solid foundation for creating effective and efficient neural networks. This initial understanding serves as a crucial stepping stone towards developing more advanced models and applications in the field of artificial intelligence.
Learn about different types of neural network architectures such as feedforward, convolutional, and recurrent neural networks
To build a strong foundation in neural networks from scratch, it is essential to explore various types of architectures. Understanding the differences and applications of feedforward, convolutional, and recurrent neural networks can provide valuable insights into how different models process data and make predictions. Feedforward networks are the simplest form, where information flows in one direction without loops. Convolutional neural networks excel at image recognition tasks by using convolutional layers to detect patterns. Recurrent neural networks are designed for sequential data processing, making them ideal for tasks like speech recognition and language translation. By delving into these diverse architectures, one can gain a comprehensive understanding of the capabilities and nuances of neural networks.
Understand the role of activation functions in neural networks
Understanding the role of activation functions in neural networks is crucial for grasping how these networks process information and make predictions. Activation functions introduce non-linearity to the network, allowing it to learn complex patterns in data. By applying an activation function to the output of each neuron, neural networks can capture intricate relationships between input variables, enabling them to model and adapt to various types of data more effectively. Different activation functions serve different purposes, influencing how information flows through the network and ultimately impacting its performance. Therefore, a solid understanding of activation functions is essential for building and training efficient neural networks from scratch.
Learn how to initialise weights and biases properly to prevent issues like vanishing or exploding gradients
To build a successful neural network from scratch, it is crucial to understand the importance of properly initializing weights and biases. By setting these parameters correctly, you can prevent common issues such as vanishing or exploding gradients. When weights are initialized too small, the gradients during backpropagation may become increasingly smaller, leading to slow learning or stagnation in training. On the other hand, if weights are initialized too large, gradients can explode, causing unstable training and difficulty in convergence. Learning how to initialize weights and biases effectively ensures a more stable and efficient neural network that can learn effectively from data.
Implement forward propagation to make predictions using the neural network
Implementing forward propagation is a crucial step in utilising a neural network to make predictions. By feeding input data through the network and calculating the activations for each layer, we can generate output predictions based on the learned parameters. This process allows us to understand how the neural network processes information and produces results, laying the foundation for further refining its performance through training and optimisation techniques.
Implement backpropagation to update weights and biases during training
Implementing backpropagation is a crucial step in training a neural network from scratch. Backpropagation allows us to calculate the gradients of the loss function with respect to the weights and biases in the network. By updating these parameters in the opposite direction of the gradient, we can iteratively improve the model’s performance during training. This process enables the neural network to learn from its mistakes and make more accurate predictions over time. Backpropagation is a fundamental concept in neural network training and plays a key role in optimising the model for better performance.
Regularise your neural network using techniques like L1/L2 regularization or dropout to prevent overfitting
To enhance the performance and generalization of your neural network built from scratch, it is crucial to incorporate regularization techniques such as L1/L2 regularization or dropout. These methods help prevent overfitting by adding penalties to the weights or randomly dropping neurons during training. Regularization encourages the network to learn essential features from the data while reducing the risk of memorizing noise or irrelevant patterns, ultimately improving its ability to make accurate predictions on unseen data.
Monitor the training process by visualising metrics like loss and accuracy on a validation set
Monitoring the training process of a neural network by visualising metrics such as loss and accuracy on a validation set is crucial for assessing the model’s performance and making informed decisions about its training. By tracking these metrics, developers can identify trends, detect overfitting or underfitting issues, and adjust hyperparameters to improve the model’s generalisation capabilities. Visualising these metrics provides valuable insights into how the neural network is learning and adapting to the data, ultimately leading to more effective model optimisation and better overall performance.
Experiment with hyperparameters like learning rate, batch size, and number of layers to optimise the performance of your neural network
To enhance the performance of your neural network built from scratch, it is recommended to experiment with various hyperparameters such as learning rate, batch size, and the number of layers. Adjusting the learning rate can impact how quickly or slowly the model learns from the data. Modifying the batch size can influence the stability and speed of convergence during training. Additionally, varying the number of layers in the network can enable you to explore different levels of complexity and abstraction in capturing patterns within the data. By optimising these hyperparameters through experimentation, you can fine-tune your neural network for improved efficiency and accuracy in handling diverse tasks and datasets.